7569번: 토마토
첫 줄에는 상자의 크기를 나타내는 두 정수 M,N과 쌓아올려지는 상자의 수를 나타내는 H가 주어진다. M은 상자의 가로 칸의 수, N은 상자의 세로 칸의 수를 나타낸다. 단, 2 ≤ M ≤ 100, 2 ≤ N ≤ 100,
www.acmicpc.net
https://onlytrying.tistory.com/229
예전에 풀었던 토마토 문제와 동일한 문제였다.
차이점은 상자의 상태가 3차원 배열이라는 점이다.
따라서, 기존의 구현 코드에서 상자를 3차원 배열로 변경하고 Z 좌표가 바뀌는 경우를 추가해주었다.
#include <iostream>
#include <cstring>
#include <queue>
using namespace std;
struct boxPos {
int z, y, x;
};
int M, N, H;
int box[101][101][101];
queue<boxPos> ripeTomatoPosQ;
int dz[6] = { 0,0,0,0,1,-1 };
int dy[6] = { 0,0,1,-1,0,0 };
int dx[6] = { 1,-1,0,0,0,0 };
int getDay(int unripeTomato) {
int day = 0;
while (unripeTomato > 0) {
day++;
int newRipeTomatoCnt = ripeTomatoPosQ.size();
// 안익은 토마토가 남아있는 상태에서 새롭게 익은 토마토가 없다면 토마토를 모두 익히는건 불가능하다.
if (newRipeTomatoCnt == 0)
return -1;
for (int i = 0; i < newRipeTomatoCnt; i++) {
int hereZ = ripeTomatoPosQ.front().z;
int hereY = ripeTomatoPosQ.front().y;
int hereX = ripeTomatoPosQ.front().x;
ripeTomatoPosQ.pop();
// 주변의 토마토를 익힌다.
for (int i = 0; i < 6; i++) {
int nearZ = hereZ + dz[i];
int nearY = hereY + dy[i];
int nearX = hereX + dx[i];
if (nearZ >= 0 && nearZ < H && nearY >= 0 && nearY < N && nearX >= 0 && nearX < M)
if (box[nearZ][nearY][nearX] == 0) {
// 안익은 토마토를 익은 토마토로 바꾸고 안익은 토마토의 갯수를 1 감소시킨다.
box[nearZ][nearY][nearX] = 1;
unripeTomato--;
// 새롭게 익은 토마토의 좌표를 큐에 담는다.
ripeTomatoPosQ.push({ nearZ, nearY, nearX });
}
}
}
}
return day;
}
int main(void) {
cin.tie(0);
ios_base::sync_with_stdio(0);
cin >> M >> N >> H;
int unripeTomato = 0;
for (int z = 0; z < H; z++) {
for (int y = 0; y < N; y++) {
for (int x = 0; x < M; x++) {
int state;
cin >> state;
box[z][y][x] = state;
if (state == 0)
unripeTomato++;
else if (state == 1)
ripeTomatoPosQ.push({ z,y,x });
}
}
}
cout << getDay(unripeTomato) << endl;
return 0;
}
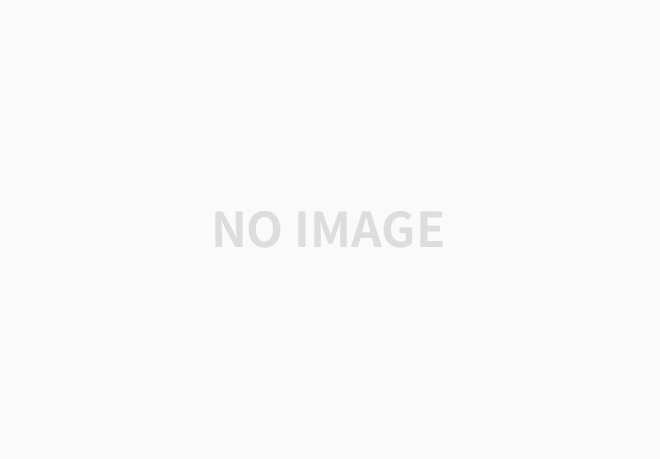
'알고리즘 > BOJ' 카테고리의 다른 글
백준 25206번: 너의 평점은 (0) | 2025.03.21 |
---|---|
백준 5430번: AC (0) | 2022.10.15 |
백준 1764번: 듣보잡 (0) | 2022.10.15 |
백준 4963번: 섬의 개수 (0) | 2022.10.15 |
백준 11724번: 연결 요소의 개수 (0) | 2022.10.15 |